Traditionally we used Multi-Page Applications (MPA) as web apps, in which a new page gets loaded from the server with each click. It not only takes time but also increases server load, slowing down the website.
AngularJS is a JavaScript-based front-end web framework that uses bidirectional UI data binding, and thus we can design Single Page Applications using AngularJS.
Single Page Applications involves loading a single HTML page and updating only a portion of the page rather than the complete page with each mouse click.
During the procedure, the page does not reload or transfer control to another page. It guarantees good performance and faster page loading.
The SPA approach is like a standard in order web applications. UI-related data from the server is delivered to the client at the start.
Only the required information is fetched from the server as the client clicks particular parts of the webpage, and the page is dynamically rewritten. This reduces the burden on the server while also saving money.
Advantages of SPA
- No page refresh: When using Single Page Application using AngularJS, you only need to load the section of the page that needs to be modified, rather than the entire page. All of your pages may be pre-loaded and cached with Angular, eliminating the need for additional requests to obtain them.
- Better user experience:Single Page Application using AngularJS has the feel of a native app, it’s quick and responsive.
- Easier Debugging: Single-page applications are easy to debug with Chrome because they are built with developer tools like AngularJS Batarang and React.
- Ability to work offline:The UI doesn’t freeze in case of loss of connectivity and can still perform error handling and displaying of appropriate messages to the user.
Step by step guide to build SPA using AngularJS:
- Module Creation: Creating a module is the first step in any AngularJS Single page application. A module is a container for the many components of your application, such as controllers, service, and so on.
var app = angular.module('myApp', []);
- Defining a Simple Controller:
app.controller('HomeController', function($scope) {
$scope.message = 'Hello from HomeController';
});
- Including AngularJS script in HTML code: We need to use module and controller in our HTML after we’ve developed them. First and foremost, we must include the angular script and app.js that we created. Then, in the ng-app attribute, we must specify our module, and in the ng-controller attribute, we must specify our controller.
<!doctype html>
<html ng-app="myApp">
<head>
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular.min.js"></script>
</head>
<body ng-controller="HomeController">
<h1>{{message}}</h1>
<script src="app.js"></script>
</body>
</html>
The output will look like this when we run the code on localhost.
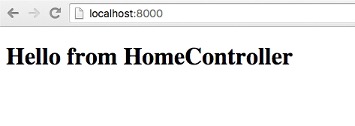
It’s now established that our module and controller are configured correctly and that AngularJS is operational.
- Using AngularJS’s routing capabilities to add different views to our SPA:
After the main angular script, we must add the angular-route script.
<script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular-route.min.js"></script>
To enable routing, we must utilize the ngRoute directive.
var app = angular.module('myApp', ['ngRoute']);
- Creating an HTML layout for the website:After we’ve generated an HTML layout for the website, we’ll use the ng-view directive to designate where the HTML for each page will be placed in the layout.
<!doctype html> <html ng-app="myApp"> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular-route.min.js"></script> </head> <body> <div ng-view></div> <script src="app.js"></script> </body> </html>
- To set the navigation to different views, use the $routeProvider service from the ngRoute module:
For each route that we want to add, we must specify a templateUrl and a controller. When a user attempts to travel to a route that does not exist, exception handling must be accommodated. We can use an “otherwise” function to redirect the user to the “/” route for simplicity.
var app = angular.module('myApp', ['ngRoute']); app.config(function($routeProvider) { $routeProvider .when('/', { templateUrl : 'pages/home.html', controller : 'HomeController' }) .when('/blog', { templateUrl : 'pages/blog.html', controller : 'BlogController' }) .when('/about', { templateUrl : 'pages/about.html', controller : 'AboutController' }) .otherwise({redirectTo: '/'}); });
- Building controllers for every specified route in $routeProvider:
For each route, we’ll need to create controllers. Controller names were set in routeProvider.
app.controller('HomeController', function($scope) { $scope.message = 'Hello from HomeController'; }); app.controller('BlogController', function($scope) { $scope.message = 'Hello from BlogController'; }); app.controller('AboutController', function($scope) { $scope.message = 'Hello from AboutController'; });
- Configuring the pages:
home.html –
<h1>Home</h1> <h3>{{message}}</h3>
blog.html –
<h1>Blog</h1> <h3>{{message}}</h3>
about.html –
<h1>About</h1> <h3>{{message}}</h3>
- Adding links to the HTML that will help in navigating to the configured pages:
<!doctype html> <html ng-app="myApp"> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular-route.min.js"></script> </head> <body> <a href="#/">Home</a> <a href="#/blog">Blog</a> <a href="#/about">About</a> <div ng-view></div> <script src="app.js"></script> </body> </html>
- Including the HTML of routing pages to index.html file using script tag:
Use the script tag with the type text/ng-template to add your partial HTML to index.html. When Angular encounters these templates, it will save their content to the template cache rather than making an Ajax request to retrieve it.
<!doctype html> <html ng-app="myApp"> <head> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.4.7/angular-route.min.js"></script> </head> <body> <script type="text/ng-template" id="pages/home.html"> <h1>Home</h1> <h3>{{message}}</h3> </script> <script type="text/ng-template" id="pages/blog.html"> <h1>Blog</h1> <h3>{{message}}</h3> </script> <script type="text/ng-template" id="pages/about.html"> <h1>About</h1> <h3>{{message}}</h3> </script> <a href="#/">Home</a> <a href="#/blog">Blog</a> <a href="#/about">About</a> <div ng-view></div> <script src="app.js"></script> </body> </html>
Output:
Once the HTML is run on localhost, the following page is displayed.
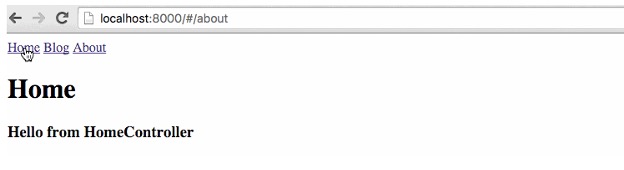
The hyperlinks First, Second, and Third on the page are routers, and when you click on them, you will be taken to the relevant web pages without having to refresh the page.
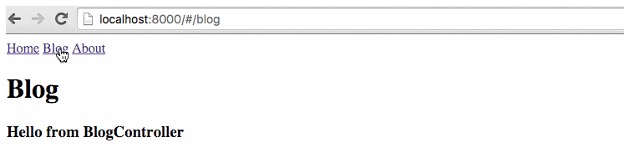
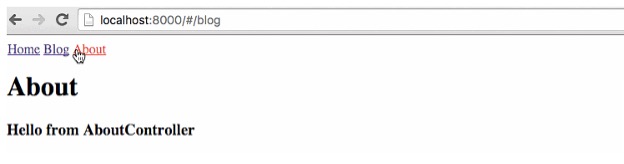
So this is all about how you can build SPA using AngularJS. If you’re working on a single-page application, AngularJS is the obvious choice.